Create Database Table and Insert records!
In this tutorial, we will walk you through the process of creating a database table and inserting records into it. Before you begin, please ensure that you have completed Tutorial 1, which covers creating the package.
Create Travel Table.
Let’s go ahead and create a database table to store the Travel data. Think of a Travel entity as a way to store all the essential information about a trip. This includes details like the agency ID, customer ID, the current status of the travel booking (whether it’s confirmed, pending, etc.), and the price of the travel. By creating this table, you’re setting up the foundation for managing travel-related data in your application.
Right-click on your ABAP package and choose New > Other ABAP Repository Object from the context menu.
Replace the default code with the provided code snippet, ensuring that you use your own table name and description in place of the defaults. This will ensure the table is correctly defined according to your naming conventions and description.
@EndUserText.label : 'Table for Travel data'
@AbapCatalog.enhancement.category : #NOT_EXTENSIBLE
@AbapCatalog.tableCategory : #TRANSPARENT
@AbapCatalog.deliveryClass : #A
@AbapCatalog.dataMaintenance : #RESTRICTED
define table zraptech_travel {
key client : abap.clnt not null;
key travel_id : /dmo/travel_id not null;
agency_id : /dmo/agency_id;
customer_id : /dmo/customer_id;
begin_date : /dmo/begin_date;
end_date : /dmo/end_date;
@Semantics.amount.currencyCode : 'zraptech_travel.currency_code'
booking_fee : /dmo/booking_fee;
@Semantics.amount.currencyCode : 'zraptech_travel.currency_code'
total_price : /dmo/total_price;
currency_code : /dmo/currency_code;
description : /dmo/description;
overall_status : /dmo/overall_status;
attachment : /dmo/attachment;
mime_type : /dmo/mime_type;
file_name : /dmo/filename;
created_by : abp_creation_user;
created_at : abp_creation_tstmpl;
local_last_changed_by : abp_locinst_lastchange_user;
local_last_changed_at : abp_locinst_lastchange_tstmpl;
last_changed_at : abp_lastchange_tstmpl;
}
Insert records in Travel table
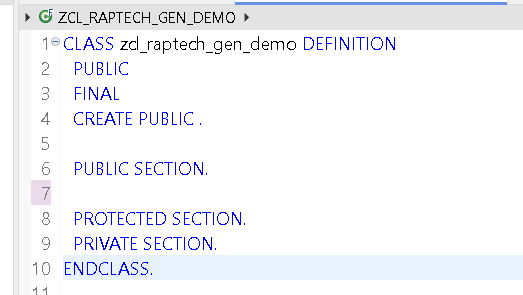
In Public section of class definition add INTERFACES if_oo_adt_classrun.
The INTERFACES if_oo_adt_classrun is used in ABAP to enable the execution of a class directly within the ABAP Development Tools (ADT) environment. It allows a class to be run in an ADT-enabled environment without the need to create an external program or report.
When a class implements the if_oo_adt_classrun interface, it must implement the main method, which serves as the entry point for execution. This is particularly useful when you want to test or execute class-based logic interactively within the ADT without needing a dedicated execution context.
Now write an insert statement after Deletion of records from the Travel table
If you save and active this, the data won’t be get inserted unless we have commit statement
CLASS zcl_raptech_gen_demo DEFINITION
PUBLIC
FINAL
CREATE PUBLIC .
PUBLIC SECTION.
INTERFACES if_oo_adt_classrun.
PROTECTED SECTION.
PRIVATE SECTION.
ENDCLASS.
CLASS zcl_raptech_gen_demo IMPLEMENTATION.
METHOD if_oo_adt_classrun~main.
" data decleration
DATA:
attachment TYPE /dmo/attachment,
file_name TYPE /dmo/filename,
mime_type TYPE /dmo/mime_type.
" clear travel table
DELETE FROM ZRAPTECH_TRAVEL.
"insert travel demo data
INSERT ZRAPTECH_TRAVEL FROM (
SELECT
FROM /dmo/travel AS travel
FIELDS
travel~travel_id AS travel_id,
travel~agency_id AS agency_id,
travel~customer_id AS customer_id,
travel~begin_date AS begin_date,
travel~end_date AS end_date,
travel~booking_fee AS booking_fee,
travel~total_price AS total_price,
travel~currency_code AS currency_code,
travel~description AS description,
CASE travel~status "[N(New) | P(Planned) | B(Booked) | X(Cancelled)]
WHEN 'N' THEN 'O'
WHEN 'P' THEN 'O'
WHEN 'B' THEN 'A'
ELSE 'X'
END AS overall_status,
@attachment AS attachment,
@mime_type AS mime_type,
@file_name AS file_name,
travel~createdby AS created_by,
travel~createdat AS created_at,
travel~lastchangedby AS last_changed_by,
travel~lastchangedat AS last_changed_at,
travel~lastchangedat AS local_last_changed_at
ORDER BY travel_id UP TO 10 ROWS
).
COMMIT WORK. " To commit in database table
out->write( |Demo data generated for table ZRAPTECH_TRAVEL.| ).
ENDMETHOD.
ENDCLASS.
To run your class, select the ABAP class, click the Run button, then choose Run As > ABAP Application (Console), or simply press F9.
Preview your table data
Open your Travel database table and press F8 to initiate the data preview and view the populated database entries (i.e., the travel data).
Congratulations! You’ve successfully completed the tutorial on creating a database table and inserting records into it. We hope this guide has helped you build a solid understanding of the process.
Feel free to explore more tutorials to expand your skills, and don’t hesitate to revisit any section if needed. Keep coding and happy learning!
Thank you for following along, and we look forward to helping you with your next steps.